Component Library 1
By Mark Foyster
JS, CSS & PHP Components
Some slightly more complicated components that use a little JavaScript alongside CSS and / or some PHP.
Contents
[MENU BAR] [POP UP WINDOW] [TELETYPE SCROLLER]
Simple Menu Bar
This is the menu bar system I am using for this website. It's nothing special but I quite like it. Here's a screensot of it:
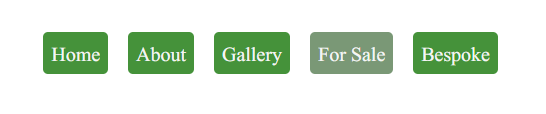
The nav bar is set in a flexbox style container set to evenly distribute the buttons horizontally and overflow will wrap. it's quite plain but customising it takes little effort.
Implementation
Simply download the files from the menu subdirectory in the GitHub Repository add the stylesheet to your `<head>` like so:
<link rel="stylesheet" href="menu/menu.css">
Insert the following PHP into the page where you wish to add the menu (eg, a header container):
<?php $_GET['id']=1; include 'menu/menu.php' ?>
You will need to change the id number to the number corresponding to the button this page links to. Finally, alter menu.php to suit. Here's an example:
<?php
function isSelected($id){
if ($_GET['id'] == $id) echo " class=\"menuItemSelected\"";
else echo " class=\"menuItemUnselected menuItemHoverable\"";
}
?>
<nav class="menuContainer">
<a href="index.php"<?php isSelected(1);?>>Home</a>
<a href="gallery.php"<?php isSelected(2);?>>Gallery</a>
</nav>
All you need to do is change the href values to your pages and ensure you have the correct id for that page as the parameter for the isSelected function.
Finally, the text between the anchor (`<a></a>`) tags is the text that will be displayed on the buttons, so alter it appropriately. Add more anchors in the same manor to add more buttons.
Customise the style
This is done using menu.css. There are custom properties for the colours like so:
:root{
--menuColor: #45923a;
--menuSelected: #7a9876;
}
Alter the colours to suit your style. Further alterations are strtaighforward by manipulating the CSS directly.
Pop Up

Press the buttons to see the popup's, code can be found in my GitHub repository HERE
Note how scrolling on the main window is disabled when the popup is active. This makes it easier to use scrolling within the popup. The down side is that when the web page has scroll bars, they will disappear while the popup is activated. The underlying screen will slightly re-size shifting the content within it to utilise the additional width.
Implementation
Make a sub directory `popup` then add `popup.js`, `popup.css` & `closebtn.png` to it then add the following html within your head:
<link rel="stylesheet" href="popup/popup.css">
<script src="popup/popup.js" defer>
Add the following html within your body:
<div class="popUp" id="popUp">
<div class="popUpTitleContainer">>span class="popUpTitle"></span></div>
<img id="closeBtn" src="popup/closebtn.png">
<div id="popUpBody"></div>
</div>
Create a file in the popup subdirectory called 'default.html' and place the html content for the popup inside it.
Call the `activatePopup()` function to launch the product. Pass the title as the first parameter. For example, you could use an onclick event handler on a button like this:
<button type="button" onclick="activatePopup('Pop Up Title Here')">Pop Up</button>
Multiple Pop Up's
The steps are as above but you simply create a different html file for each Pop Up within the 'popup' subdirectory and pass the name (minus the '.html') as the second parameter when you call the function. Here's an example, in this case the contents is in 'newfile.html':
NOTE: You CANNOT choose 'override' as a name for an external html file. It's reserved to allow the loading of external html to be overriden. For an example of this, check out the GALLERY project.
<button type="button" onclick="activatePopup('New Title Here','newfile')">Pop Up</button>
There is no real limit to how many pop up's you can have, it's just one pop up and the contents is loaded dynamically. Because of this, only one pop up can be displayed at a time, they cannot be overlayed. It would be possible to change the contents from within the pop up by simply calling 'activatePopup' twice. The first time would close the pop up and the second is where you would pass your new title and content filename as parameters to re-invoke it. I haven't had a need to try this yet, so this idea is untested!
Customising style
Within the popup.css, at the top are the following custom properties:
:root{
/* COLOURS */
--popUpBackgroundColour: whitesmoke;
--popUpBoxShadowColour: rgb(141, 139, 139); /* Best to set this a darker tone of the background colour */
--popUpTitleColour:red;
--popUpBorderColour: mediumblue;
/* SIZES */
--popUpSize: 90%; /*This is the size of the entire popup relative to screen*/
--popUpTitleFontSize: 2rem;
}
Simply change the colours and sizes to your preference for minor changes.The remainder of this CSS file is pretty basic. Further customisation is easy using fundamental CSS knowledge.
TTY Heading
This is my TTY Heading, hover over me!
This is not terribly practical but I had a lot of fun making it.
This one was for fun. I used it for an early portfolio website I made a few months back. It's not terribly practical butI had a lot of fun with it. The font is a 'fixed pitch' variety (VT323) I found on Google Fonts. The license is in a text file along with the font in the 'font' subdirectory in this component's repo.
The code can be found in my GitHub repository HERE. Have fun!